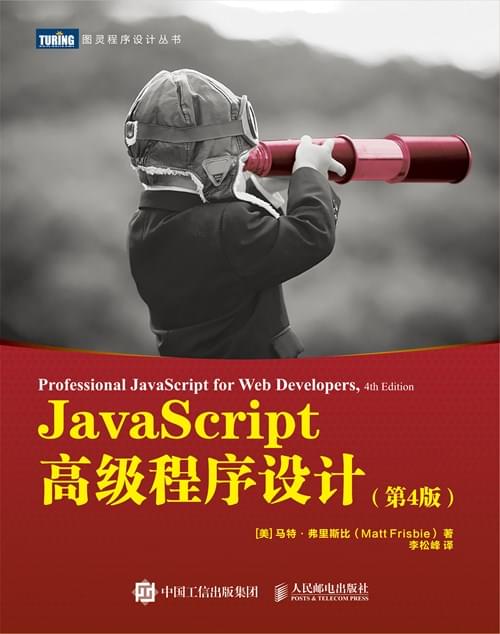
2024-12-22 日报 Day43
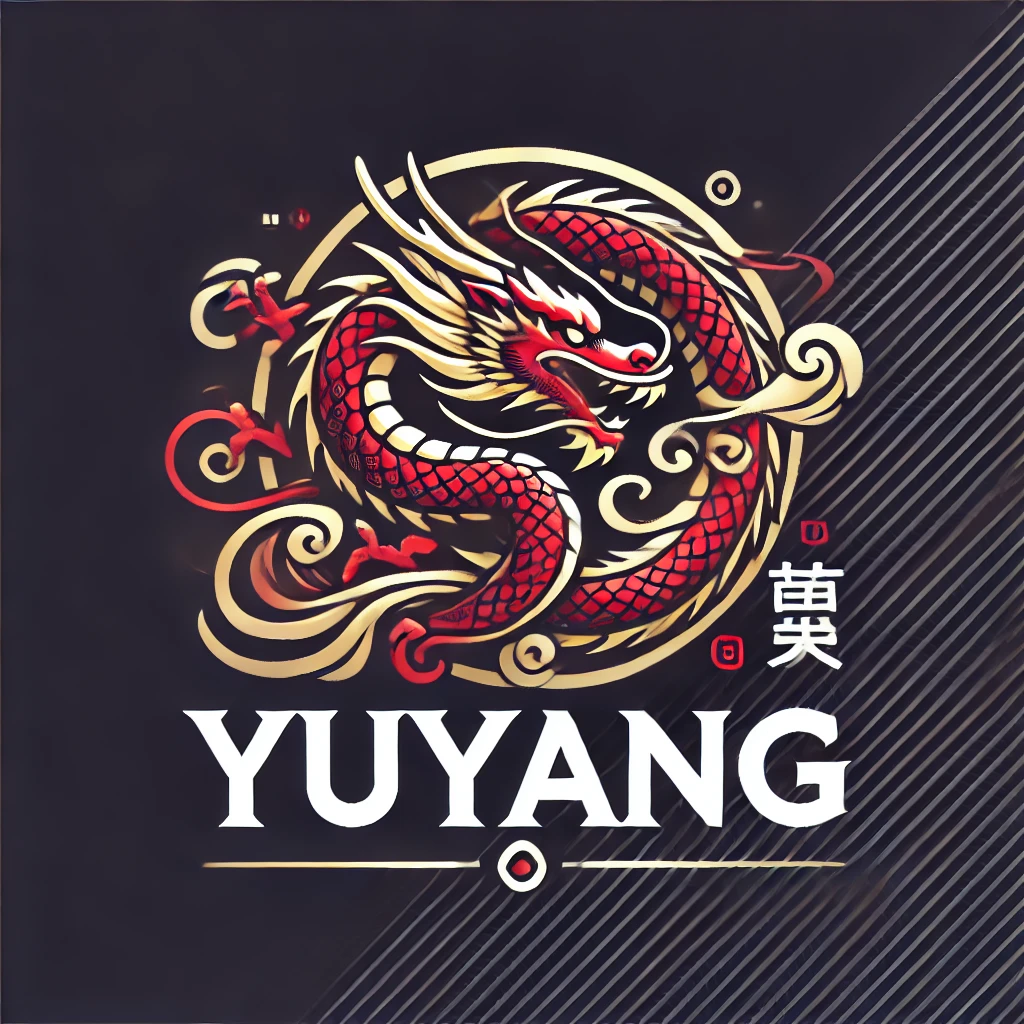
今日的鸡汤
成功其实很简单,就是比失败击倒你们的次数多一次。
今日学习内容
1、JS红皮书P120-124 第五章:基本引用类型
今日笔记
1、某些 Unicode 字符可以有多种编码方式。有的字符既可以通过一个 BMP 字符表示,也可以通过一个代理对表示。比如:
1 | // U+00C5:上面带圆圈的大写拉丁字母 A |
为解决这个问题,Unicode提供了 4种规范化形式,可以将类似上面的字符规范化为一致的格式,无论底层字符的代码是什么。这 4种规范化形式是:NFD(Normalization Form D)、NFC(Normalization Form C)、NFKD(Normalization Form KD)和 NFKC(Normalization Form KC)。可以使用 normalize()方法对字符串应用上述规范化形式,使用时需要传入表示哪种形式的字符串:”NFD”、”NFC”、”NFKD”或”NFKC”。
2、字符串操作方法:
- concat():将一个或多个字符串拼接起来,返回拼接后的新字符串。
1
2
3
4let stringValue = "hello ";
let result = stringValue.concat("world");
console.log(result); // "hello world"
console.log(stringValue); // "hello" - slice():提取字符串的一部分,返回一个新字符串,不会改变原始字符串。
1
2
3
4
5
6
7let stringValue = "hello world";
console.log(stringValue.slice(3)); // "lo world"
console.log(stringValue.substring(3)); // "lo world"
console.log(stringValue.substr(3)); // "lo world"
console.log(stringValue.slice(3, 7)); // "lo w"
console.log(stringValue.substring(3,7)); // "lo w"
console.log(stringValue.substr(3, 7)); // "lo worl" - substr():从字符串中提取指定数目的字符,返回新字符串。
- substring():提取字符串中两个指定的索引位置之间的字符,返回新字符串。
slice()、substr()和 substring()是以相同方式被调用的,而且多数情况下返
回的值也相同。如果只传一个参数 3,则所有方法都将返回”lo world”,因为”hello”中”l”位置为 3。如果传入两个参数 3 和 7,则 slice()和 substring()返回”lo w”(因为”world”中”o”在位置 7,不包含),而 substr()返回”lo worl”,因为第二个参数对它而言表示返回的字符数。
当某个参数是负值时,这 3 个方法的行为又有不同。比如,slice()方法将所有负值参数都当成字符串长度加上负参数值。在给 slice()和 substr()传入负参数时,它们的返回结果相同。这是因为-3 会被转换为 8(长度加上负参数),实际上调用的是 slice(8)和 substr(8)。而substring()方法返回整个字符串,因为-3 会转换为 0。在第二个参数是负值时,这 3 个方法各不相同。slice()方法将第二个参数转换为 7,实际上相当于调用 slice(3, 7),因此返回”lo w”。而 substring()方法会将第二个参数转换为 0,相当于调用substring(3, 0),等价于 substring(0, 3),这是因为这个方法会将较小的参数作为起点,将较大的参数作为终点。对 substr()来说,第二个参数会被转换为 0,意味着返回的字符串包含零个字符,因而会返回一个空字符串。1
2
3
4
5
6
7let stringValue = "hello world";
console.log(stringValue.slice(-3)); // "rld"
console.log(stringValue.substring(-3)); // "hello world"
console.log(stringValue.substr(-3)); // "rld"
console.log(stringValue.slice(3, -4)); // "lo w"
console.log(stringValue.substring(3, -4)); // "hel"
console.log(stringValue.substr(3, -4)); // "" (empty string) - 字符串位置方法:indexOf()、lastIndexOf()。这两个方法从字符串中搜索传入的字符串,并返回位置(如果没找到,则返回-1)。两者的区别在于,indexOf()方法从字符串开头开始查找子字符串,而 lastIndexOf()方法从字符串末尾开始查找子字符串。这两个方法都可以接收可选的第二个参数,表示开始搜索的位置。这意味着,indexOf()会从这个参数指定的位置开始向字符串末尾搜索,忽略该位置之前的字符;lastIndexOf()则会从这个参数指定的位置开始向字符串开头搜索,忽略该位置之后直到字符串末尾的字符。下面看一个例子:
1
2
3let stringValue = "hello world";
console.log(stringValue.indexOf("o")); // 4
console.log(stringValue.lastIndexOf("o")); // 7在传入第二个参数 6 以后,结果跟前面的例子恰好相反。这一次,indexOf()返回 7,因为它从位置 6(字符”w”)开始向后搜索字符串,在位置 7 找到了”o”。而 lastIndexOf()返回 4,因为它从位置 6 开始反向搜索至字符串开头,因此找到了”hello”中的”o”。1
2
3let stringValue = "hello world";
console.log(stringValue.indexOf("o", 6)); // 7
console.log(stringValue.lastIndexOf("o", 6)); // 4 - 字符串包含方法:startsWith()、endsWith()和 includes()。这些方法都会从字符串中搜索传入的字符串,并返回一个表示是否包含的布尔值。它们的区别在于,startsWith()检查开始于索引 0 的匹配项,endsWith()检查开始于索引(string.length - substring.length)的匹配项,而 includes()检查整个字符串:startsWith()和 includes()方法接收可选的第二个参数,表示开始搜索的位置。如果传入第二个参数,则意味着这两个方法会从指定位置向着字符串末尾搜索,忽略该位置之前的所有字符。下面是一个例子:
1
2
3
4
5
6
7
8
9let message = "foobarbaz";
console.log(message.startsWith("foo")); // true
console.log(message.startsWith("bar")); // false
console.log(message.endsWith("baz")); // true
console.log(message.endsWith("bar")); // false
console.log(message.includes("bar")); // true
console.log(message.includes("qux")); // falseendsWith()方法接收可选的第二个参数,表示应该当作字符串末尾的位置。如果不提供这个参数,那么默认就是字符串长度。如果提供这个参数,那么就好像字符串只有那么多字符一样:1
2
3
4
5let message = "foobarbaz";
console.log(message.startsWith("foo")); // true
console.log(message.startsWith("foo", 1)); // false
console.log(message.includes("bar")); // true
console.log(message.includes("bar", 4)); // false1
2
3let message = "foobarbaz";
console.log(message.endsWith("bar")); // false
console.log(message.endsWith("bar", 6)); // true - trim():创建一个字符串的副本,删除前置及后缀的所有空格,然后返回结果。由于 trim()返回的是字符串的副本,因此原始字符串不受影响,即原本的前、后空格符都会保留。另外,trimLeft()和 trimRight()方法分别用于从字符串开始和末尾清理空格符。
1
2
3
4let stringValue = " hello world ";
let trimmedStringValue = stringValue.trim();
console.log(stringValue); // " hello world "
console.log(trimmedStringValue); // "hello world" - repeat():ECMAScript 在所有字符串上都提供了 repeat()方法。这个方法接收一个整数参数,表示要将字符串复制多少次,然后返回拼接所有副本后的结果。
1
2
3let stringValue = "na ";
console.log(stringValue.repeat(16) + "batman");
// na na na na na na na na na na na na na na na na batman - padStart()和 padEnd():padStart()和 padEnd()方法会复制字符串,如果小于指定长度,则在相应一边填充字符,直至满足长度条件。这两个方法的第一个参数是长度,第二个参数是可选的填充字符串,默认为空格(U+0020)。可选的第二个参数并不限于一个字符。如果提供了多个字符的字符串,则会将其拼接并截断以匹配指定长度。此外,如果长度小于或等于字符串长度,则会返回原始字符串。
1
2
3
4
5let stringValue = "foo";
console.log(stringValue.padStart(6)); // " foo"
console.log(stringValue.padStart(9, ".")); // "......foo"
console.log(stringValue.padEnd(6)); // "foo "
console.log(stringValue.padEnd(9, ".")); // "foo......"1
2
3
4
5let stringValue = "foo";
console.log(stringValue.padStart(8, "bar")); // "barbafoo"
console.log(stringValue.padStart(2)); // "foo"
console.log(stringValue.padEnd(8, "bar")); // "foobarba"
console.log(stringValue.padEnd(2)); // "foo" - 字符串迭代与解构:字符串的原型上暴露了一个@@iterator 方法,表示可以迭代字符串的每个字符。可以像下面这样手动使用迭代器:在 for-of 循环中可以通过这个迭代器按序访问每个字符:
1
2
3
4
5
6let message = "abc";
let stringIterator = message[Symbol.iterator]();
console.log(stringIterator.next()); // {value: "a", done: false}
console.log(stringIterator.next()); // {value: "b", done: false}
console.log(stringIterator.next()); // {value: "c", done: false}
console.log(stringIterator.next()); // {value: undefined, done: true}有了这个迭代器之后,字符串就可以通过解构操作符来解构了。比如,可以更方便地把字符串分割为字符数组:1
2
3
4
5
6
7
8for (const c of "abcde") {
console.log(c);
}
// a
// b
// c
// d
// e1
2let message = "abcde";
console.log([...message]); // ["a", "b", "c", "d", "e"] - 字符串大小写转换:下一组方法涉及大小写转换,包括 4 个方法:toLowerCase()、toLocaleLowerCase()、toUpperCase()和toLocaleUpperCase()。toLowerCase()和toUpperCase()方法是原来就有的方法,与 java.lang.String 中的方法同名。toLocaleLowerCase()和 toLocaleUpperCase()方法旨在基于特定地区实现。在很多地区,地区特定的方法与通用的方法是一样的。但在少数语言中(如土耳其语),Unicode 大小写转换需应用特殊规则,要使用地区特定的方法才能实现正确转换。下面是几个例子:
let stringValue = “hello world”;
console.log(stringValue.toLocaleUpperCase()); // “HELLO WORLD”
console.log(stringValue.toUpperCase()); // “HELLO WORLD”
console.log(stringValue.toLocaleLowerCase()); // “hello world”
console.log(stringValue.toLowerCase()); // “hello world”
这里,toLowerCase()和 toLocaleLowerCase()都返回 hello world,而 toUpperCase()和toLocaleUpperCase()都返回 HELLO WORLD。通常,如果不知道代码涉及什么语言,则最好使用地区特定的转换方法。
好嘞!我们来看看 土耳其语(Turkish) 是为什么要使用 toLocaleLowerCase() 和 toLocaleUpperCase() 的经典示例语言,以及它和普通 toLowerCase()/toUpperCase() 的区别。
⸻
🇹🇷 土耳其语大小写转换的特别之处
在英文中:
• ‘i’ → ‘I’
• ‘I’ → ‘i’
这是我们熟悉的大小写规则。
但在 土耳其语 中:
• 小写 ‘i’ → 大写 ‘İ’(带点的大写 i)
• 大写 ‘I’ → 小写 ‘ı’(不带点的小写 i)
也就是说,土耳其语有两个不同的字母:
• ‘i’(点的 i)
• ‘ı’(无点的 i)
⸻
✅ 举个代码例子:
let str = “i”;
console.log(str.toUpperCase()); // “I” —— 英文规则
console.log(str.toLocaleUpperCase(“tr-TR”)); // “İ” —— 土耳其语规则
let str2 = “I”;
console.log(str2.toLowerCase()); // “i” —— 英文规则
console.log(str2.toLocaleLowerCase(“tr-TR”)); // “ı” —— 土耳其语规则
⸻
📌 总结建议:
• 如果你处理的是英文或默认语言环境,toUpperCase() 和 toLowerCase() 足够。
• 如果你处理的是用户可能使用土耳其语、立陶宛语等语言的多语言应用,请使用 toLocaleUpperCase(locale) 和 toLocaleLowerCase(locale) 并显式指定区域,这样才能保证转换符合语言规则。
⸻